How to Add an Image Slider in Blogger (Using HTML, CSS & JS)
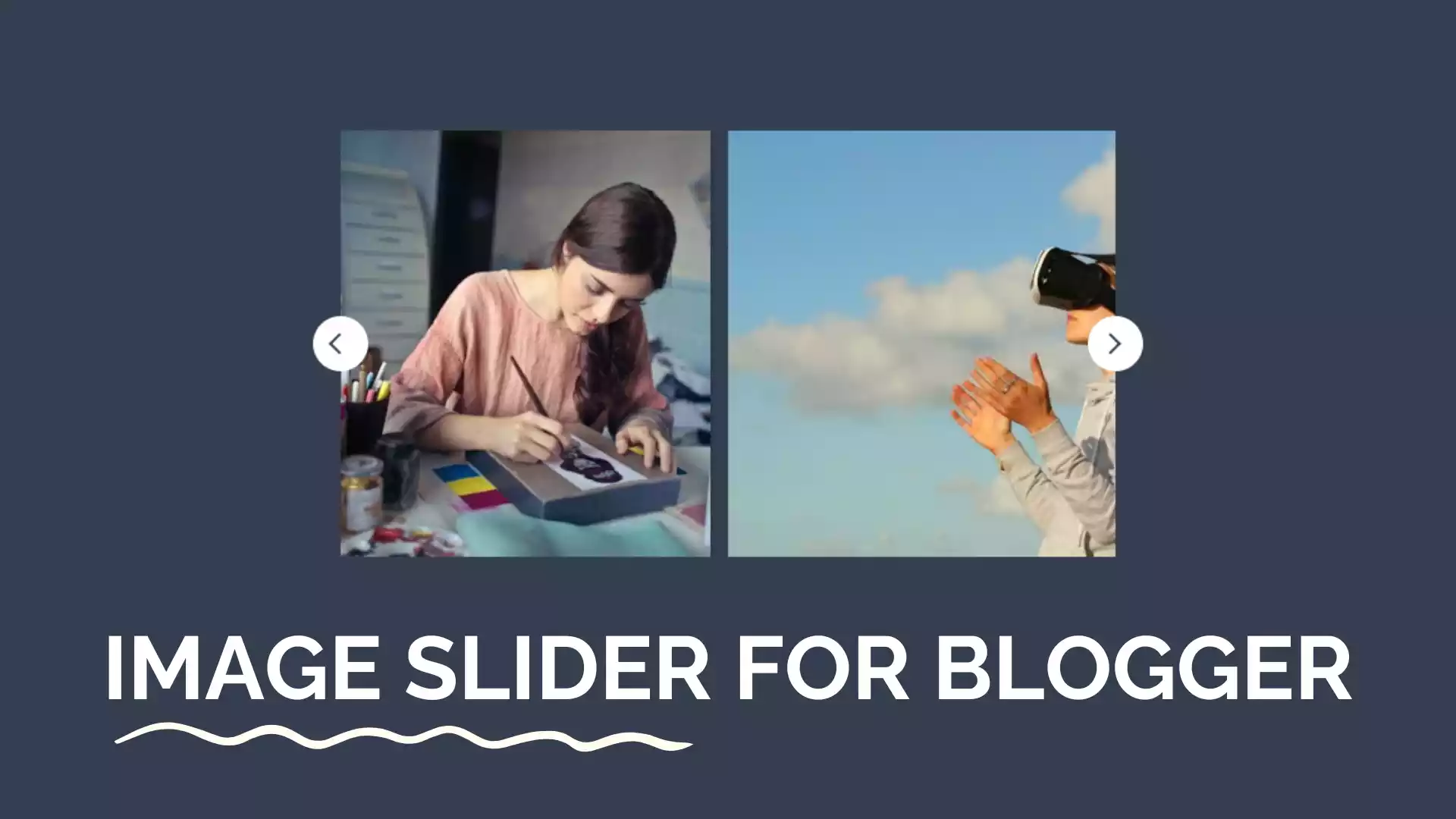
An image slider is a great way to make your Blogger website visually appealing and interactive. Whether you want to showcase images, display featured posts, or create an engaging homepage, a slider can do the job.
In this tutorial, weโll walk you through the steps to add an image slider to your Blogger website using simple HTML, CSS, and JavaScript.
- Go to the Layout section.
- Click Add a Gadget in the area where you want to add the slider (e.g., your homepage).
- Choose the HTML/JavaScript gadget.
Image Slider 1
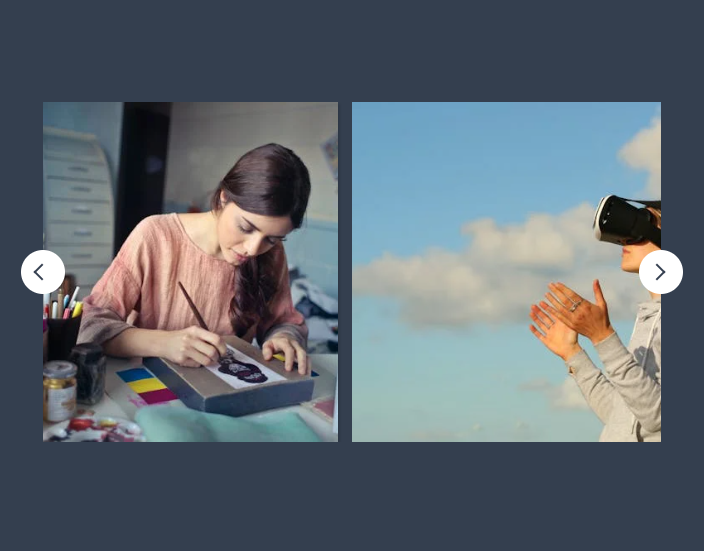
First of all, You need to Upload all images in same size and aspect ratio and Copy all image Source URLs in some notepad files.
Below is the code you need to copy and paste into the HTML/JavaScript gadget. Make sure to change the Image URLs here.
<div class="wrapper"> <div id="left" class="arrow prev"></div> <div class="carousel"> <img src="https://images.pexels.com/photos/3561009/pexels-photo-3561009.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img src="https://images.pexels.com/photos/4348404/pexels-photo-4348404.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img src="https://images.pexels.com/photos/933255/pexels-photo-933255.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img src="https://images.pexels.com/photos/123335/pexels-photo-123335.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> </div> <div id="right" class="arrow next"></div> </div> <style> .wrapper { display: flex; max-width: 1200px; position: relative; } .arrow { top: 50%; height: 44px; width: 44px; background: #fff; cursor: pointer; position: absolute; transform: translateY(-50%); border-radius: 50%; transition: transform 0.1s linear; align-content: center; } .arrow.prev { left: -22px; display: none; } .arrow.next { right: -22px; } .arrow:before { content: ""; display: inline-block; width: 10px; height: 10px; border-top: 2px solid #343F4F; border-right: 2px solid #343F4F; margin-left: 12; } .arrow.prev:before { transform: rotate(-135deg); margin-left: 15; } .arrow.next:before { transform: rotate(45deg); margin-right: 4px; } .arrow:hover { background: #f2f2f2; } .arrow:active { transform: translateY(-50%) scale(0.9); } .wrapper .carousel { font-size: 0px; cursor: pointer; overflow: hidden; white-space: nowrap; scroll-behavior: smooth; } .carousel.dragging { cursor: grab; scroll-behavior: auto; } .carousel.dragging img { pointer-events: none; } .carousel img { height: 340px; object-fit: cover; user-select: none; margin-left: 14px; width: calc(100% / 3); box-shadow: rgba(0, 0, 0, 0.15) 1.95px 1.95px 2.6px; } .carousel img:first-child { margin-left: 0px; } @media screen and (max-width: 900px) { .carousel img { width: calc(100% / 2); } } @media screen and (max-width: 550px) { .carousel img { width: 100%; } } </style> <script> const carousel = document.querySelector(".carousel"), firstImg = carousel.querySelectorAll("img")[0], arrowIcons = document.querySelectorAll(".wrapper .arrow"); let isDragStart = false, isDragging = false, prevPageX, prevScrollLeft, positionDiff; const showHideIcons = () => { // Show or hide arrows based on the scroll position let scrollWidth = carousel.scrollWidth - carousel.clientWidth; // Max scrollable width arrowIcons[0].style.display = carousel.scrollLeft == 0 ? "none" : "block"; arrowIcons[1].style.display = carousel.scrollLeft == scrollWidth ? "none" : "block"; }; arrowIcons.forEach(icon => { icon.addEventListener("click", () => { let firstImgWidth = firstImg.clientWidth + 14; // Get image width + margin // Scroll left or right based on button click carousel.scrollLeft += icon.classList.contains("prev") ? -firstImgWidth : firstImgWidth; setTimeout(() => showHideIcons(), 60); // Update arrow visibility after scroll }); }); const autoSlide = () => { // If at either end, stop auto-slide if (carousel.scrollLeft - (carousel.scrollWidth - carousel.clientWidth) > -1 || carousel.scrollLeft <= 0) return; positionDiff = Math.abs(positionDiff); // Absolute value let firstImgWidth = firstImg.clientWidth + 14; let valDifference = firstImgWidth - positionDiff; if (carousel.scrollLeft > prevScrollLeft) { // Scrolling right carousel.scrollLeft += positionDiff > firstImgWidth / 3 ? valDifference : -positionDiff; } else { // Scrolling left carousel.scrollLeft -= positionDiff > firstImgWidth / 3 ? valDifference : -positionDiff; } }; const dragStart = (e) => { isDragStart = true; prevPageX = e.pageX || e.touches[0].pageX; prevScrollLeft = carousel.scrollLeft; }; const dragging = (e) => { if (!isDragStart) return; e.preventDefault(); isDragging = true; carousel.classList.add("dragging"); positionDiff = (e.pageX || e.touches[0].pageX) - prevPageX; carousel.scrollLeft = prevScrollLeft - positionDiff; showHideIcons(); }; const dragStop = () => { isDragStart = false; carousel.classList.remove("dragging"); if (!isDragging) return; isDragging = false; autoSlide(); }; carousel.addEventListener("mousedown", dragStart); carousel.addEventListener("touchstart", dragStart); document.addEventListener("mousemove", dragging); carousel.addEventListener("touchmove", dragging); document.addEventListener("mouseup", dragStop); carousel.addEventListener("touchend", dragStop); // Initialize icons visibility showHideIcons(); </script>
After pasting the code, click the Save button. View your blog to see the slider in action.
Image slider with Auto Scroll Enabled
<div class="wrapper"> <div id="left" class="arrow prev aria-label="Previous Slide""></div> <div class="carousel"> <img src="https://images.pexels.com/photos/3561009/pexels-photo-3561009.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img src="https://images.pexels.com/photos/4348404/pexels-photo-4348404.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img loading="lazy" src="https://images.pexels.com/photos/933255/pexels-photo-933255.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> <img loading="lazy" src="https://images.pexels.com/photos/123335/pexels-photo-123335.jpeg?auto=compress&cs=tinysrgb&w=600" alt="img" draggable="false"> </div> <div id="right" class="arrow next" aria-label="Next Slide"></div> </div> <style> .wrapper { display: flex; max-width: 1200px; position: relative; } .arrow { top: 50%; height: 44px; width: 44px; background: #fff; cursor: pointer; position: absolute; transform: translateY(-50%); border-radius: 50%; transition: transform 0.1s linear; align-content: center; } .arrow.prev { left: -22px; display: none; } .arrow.next { right: -22px; } .arrow:before { content: ""; display: inline-block; width: 10px; height: 10px; border-top: 2px solid #343F4F; border-right: 2px solid #343F4F; margin-left: 12; } .arrow.prev:before { transform: rotate(-135deg); margin-left: 15; } .arrow.next:before { transform: rotate(45deg); margin-right: 4px; } .arrow:hover { background: #f2f2f2; } .arrow:active { transform: translateY(-50%) scale(0.9); } .wrapper .carousel { font-size: 0px; cursor: pointer; overflow: hidden; white-space: nowrap; scroll-behavior: smooth; } .carousel.dragging { cursor: grab; scroll-behavior: auto; } .carousel.dragging img { pointer-events: none; } .carousel img { height: 340px; object-fit: cover; user-select: none; margin-left: 14px; width: calc(100% / 3;); box-shadow: rgba(0, 0, 0, 0.15) 1.95px 1.95px 2.6px; } .carousel img:first-child { margin-left: 0px; } @media screen and (max-width: 900px) { .carousel img { width: calc(100% / 2); } } @media screen and (max-width: 550px) { .carousel img { width: 100%; } } </style> <script> const carousel = document.querySelector(".carousel"), firstImg = carousel.querySelectorAll("img")[0], arrowIcons = document.querySelectorAll(".wrapper .arrow"); let isDragStart = false, isDragging = false, prevPageX, prevScrollLeft, positionDiff; let autoScrollInterval; // Show or hide arrows based on scroll position const showHideIcons = () => { let scrollWidth = carousel.scrollWidth - carousel.clientWidth; arrowIcons[0].style.display = carousel.scrollLeft == 0 ? "none" : "block"; arrowIcons[1].style.display = carousel.scrollLeft == scrollWidth ? "none" : "block"; }; // Function to start auto-scrolling const startAutoScroll = () => { autoScrollInterval = setInterval(() => { let firstImgWidth = firstImg.clientWidth + 14; carousel.scrollLeft += firstImgWidth; if (carousel.scrollLeft >= carousel.scrollWidth - carousel.clientWidth) { carousel.scrollLeft = 0; // Reset to start when reaching the end } showHideIcons(); }, 3000); // Change slides every 3 seconds }; // Stop auto-scrolling on user interaction const stopAutoScroll = () => { clearInterval(autoScrollInterval); }; // Call the startAutoScroll function startAutoScroll(); arrowIcons.forEach(icon => { icon.addEventListener("click", () => { stopAutoScroll(); let firstImgWidth = firstImg.clientWidth + 14; carousel.scrollLeft += icon.classList.contains("prev") ? -firstImgWidth : firstImgWidth; setTimeout(() => showHideIcons(), 60); startAutoScroll(); // Resume auto-scroll after manual scroll }); }); const autoSlide = () => { if (carousel.scrollLeft - (carousel.scrollWidth - carousel.clientWidth) > -1 || carousel.scrollLeft <= 0) return; positionDiff = Math.abs(positionDiff); let firstImgWidth = firstImg.clientWidth + 14; let valDifference = firstImgWidth - positionDiff; if (carousel.scrollLeft > prevScrollLeft) { carousel.scrollLeft += positionDiff > firstImgWidth / 3 ? valDifference : -positionDiff; } else { carousel.scrollLeft -= positionDiff > firstImgWidth / 3 ? valDifference : -positionDiff; } }; const dragStart = (e) => { stopAutoScroll(); isDragStart = true; prevPageX = e.pageX || e.touches[0].pageX; prevScrollLeft = carousel.scrollLeft; }; const dragging = (e) => { if (!isDragStart) return; e.preventDefault(); isDragging = true; carousel.classList.add("dragging"); positionDiff = (e.pageX || e.touches[0].pageX) - prevPageX; carousel.scrollLeft = prevScrollLeft - positionDiff; showHideIcons(); }; const dragStop = () => { isDragStart = false; carousel.classList.remove("dragging"); if (!isDragging) return; isDragging = false; autoSlide(); startAutoScroll(); // Resume auto-scroll after drag }; carousel.addEventListener("mousedown", dragStart); carousel.addEventListener("touchstart", dragStart); document.addEventListener("mousemove", dragging); carousel.addEventListener("touchmove", dragging); document.addEventListener("mouseup", dragStop); carousel.addEventListener("touchend", dragStop); showHideIcons(); </script>
Image slider 2
You can use the below code to create an image slider like this as shown in the below screenshot.
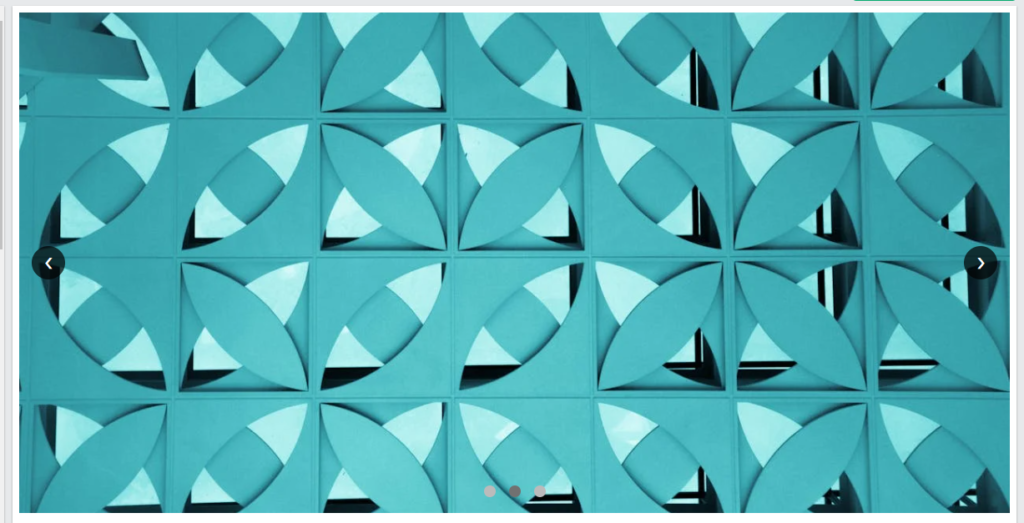
<style> /* Slider Container */ .slider-container { position: relative; max-width: 100%; height: 600px; /* Fixed height for slider */ overflow: hidden; } /* Slides */ .slide { display: none; width: 100%; height: 100%; position: relative; } .slide a img { width: 100%; height: 100%; object-fit: cover; } .prev, .next { position: absolute; top: 50%; font-size: 18px; color: #fff; background-color: rgba(0, 0, 0, 0.7); width: 40px; height: 40px; display: flex; align-items: center; justify-content: center; cursor: pointer; border-radius: 50%; transform: translateY(-50%); transition: background-color 0.3s; user-select: none; z-index: 10; } .prev:hover, .next:hover { background-color: rgba(0, 0, 0, 0.9); } .prev {left: 15px;} .next {right: 15px;} .dots { text-align: center; position: absolute; bottom: 15px; width: 100%; z-index: 10; } .dot { height: 14px; width: 14px; margin: 0 6px; background-color: #bbb; border-radius: 50%; display: inline-block; cursor: pointer; transition: background-color 0.3s; } .active, .dot:hover { background-color: #717171; } /* Responsive Styles for Mobile */ @media (max-width: 768px) { .prev, .next { width: 30px; height: 30px; font-size: 14px; } .slider-container { height: 350px; /* Fixed height for Mobile */ } } </style> <div class="slider-container"> <!-- Slide 1 --> <div class="slide"> <a href="https://example.com/page1"><img src="https://images.pexels.com/photos/414974/pexels-photo-414974.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1" alt="Slide 1"></a> </div> <!-- Slide 2 --> <div class="slide"> <a href="https://example.com/page2"><img src="https://images.pexels.com/photos/325649/pexels-photo-325649.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1" alt="Slide 2"></a> </div> <!-- Slide 3 --> <div class="slide"> <a href="https://example.com/page3"><img src="https://images.pexels.com/photos/572056/pexels-photo-572056.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1" alt="Slide 3"></a> </div> <!-- Navigation Buttons --> <a class="prev" onclick="plusSlides(-1)">❮</a> <a class="next" onclick="plusSlides(1)">❯</a> <!-- Dots --> <div class="dots"> <span class="dot" onclick="currentSlide(1)"></span> <span class="dot" onclick="currentSlide(2)"></span> <span class="dot" onclick="currentSlide(3)"></span> </div> </div> <script> // JavaScript for Slider let slideIndex = 1; showSlides(slideIndex); function plusSlides(n) { showSlides(slideIndex += n); } function currentSlide(n) { showSlides(slideIndex = n); } function showSlides(n) { let slides = document.getElementsByClassName("slide"); let dots = document.getElementsByClassName("dot"); if (n > slides.length) { slideIndex = 1 } if (n < 1) { slideIndex = slides.length } for (let i = 0; i < slides.length; i++) { slides[i].style.display = "none"; } for (let i = 0; i < dots.length; i++) { dots[i].className = dots[i].className.replace(" active", ""); } slides[slideIndex - 1].style.display = "block"; dots[slideIndex - 1].className += " active"; } // Auto Slide Functionality setInterval(function() { plusSlides(1); }, 5000); // Change slide every 5 seconds </script>
Conclusion
Adding an image slider to your Blogger website is simple and enhances its visual appeal. With just HTML, CSS, and JavaScript, you can create a fully functional and responsive slider. Now, you can showcase your content in style and keep your visitors engaged!
Don’t forget to Join Our Telegram Group for Regular updates.
โ๏ธMore: How to Hide Featured Thumbnail Image from Blogger Post
If you found this guide helpful, feel free to share it with others. Happy blogging! ๐
Thank you so much…